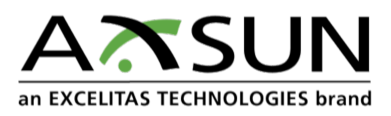 |
AxsunOCTControl_LW
1.0.0.0
A light-weight & cross-platform alternative to the .NET-based AxsunOCTControl.dll.
|
Go to the documentation of this file.
10 #ifndef AXSUNOCTCONTROLLW_C_H
11 #define AXSUNOCTCONTROLLW_C_H
15 #ifdef LabVIEW_CALLBACK
217 AxErr axGetFPGARegisterRange(
const uint32_t start_regnum,
const uint32_t end_regnum, uint16_t* regvals,
const uint32_t bytes_allocated, uint32_t which_DAQ);
309 AxErr axSetFPGADataArray(
const uint32_t regnum,
const uint16_t* data_array,
const uint32_t number_of_words, uint32_t which_DAQ);
343 AxErr axDebugCommand(uint32_t command_number, uint32_t value_out, uint32_t* command_in, uint32_t* value_in, uint32_t which_device);
367 AxErr axFPGAVersion(uint32_t* major, uint32_t* minor, uint32_t* patch, uint32_t* build, uint32_t which_device);
440 AxErr axGetIntSetting(uint32_t which_setting, int32_t* setting_value,
char * setting_string, uint32_t which_device);
452 AxErr axGetBoolSetting(uint32_t which_setting, uint8_t* setting_value,
char * setting_string, uint32_t which_device);
467 AxErr axSettingsToDevice(uint32_t float_count, uint32_t int_count, uint32_t bool_count,
float* float_values, int32_t* int_values, uint8_t* bool_values, uint32_t which_device);
590 int32_t* error_from_last_home, uint32_t* last_move_time,
591 uint8_t* state, uint8_t* home_switch, uint8_t* limit_switch, uint8_t* VDL_error, uint32_t which_laser);
620 uint32_t timer1duration, uint32_t timer2duration, uint16_t* volts_currents, uint32_t save_to_flash, uint32_t which_laser);
637 uint32_t* timer1duration, uint32_t* timer2duration, uint16_t* volts_currents, uint32_t which_laser);
669 #ifdef LabVIEW_CALLBACK
682 #endif // __cplusplus
AxErr axSetFPGARegisterSingleByte(const uint32_t regnum, const uint32_t which_byte, const uint32_t value, uint32_t which_DAQ)
Sets (i.e. writes) the low or high byte of an FPGA register, leaving the other byte unchanged.
AxErr axSettingsToDevice(uint32_t float_count, uint32_t int_count, uint32_t bool_count, float *float_values, int32_t *int_values, uint8_t *bool_values, uint32_t which_device)
Write device settings to non-volatile memory (i.e. flash).
AxErr axRegisterConnectCallback(AxConnectCallbackFunctionC_t callback_function, void *userData)
Registers a callback function to be executed following a device connection or disconnection event.
@ LVCMOS
Definition: AxsunOCTControl_LW_C.h:31
@ EXTERNAL
Definition: AxsunOCTControl_LW_C.h:28
AxErr axOpenAxsunOCTControl(uint32_t open_all_interfaces)
Opens the AxsunOCTControl context for subsequent device communication.
AxErr axSetFPGARegisterSingleNibble(const uint32_t regnum, const uint32_t which_nibble, const uint32_t value, uint32_t which_DAQ)
Sets (i.e. writes) one nibble (4 bits) of an FPGA register, leaving the other 12 bits unchanged.
@ TEC_UNINITIALIZED
Definition: AxsunOCTControl_LW_C.h:51
AxErr axSetFPGARegister(const uint32_t regnum, const uint16_t regval, uint32_t which_DAQ)
Sets (i.e. writes) a FPGA register with a single value.
AxErr axUSBInterfaceOpen(uint32_t interface_status)
Opens, resets, or closes the USB interface.
AxErr axGetFPGARegister(const uint32_t regnum, uint16_t *regval, uint32_t which_DAQ)
Gets (i.e. reads) the current value in a FPGA register.
AxErr axSerialInterfaceOpen(uint32_t interface_status, char *port)
Opens, resets, or closes a RS-232 serial interface on a given port.
AxErr axGetIntSetting(uint32_t which_setting, int32_t *setting_value, char *setting_string, uint32_t which_device)
Gets a integer setting's value and name string.
@ USB
Definition: AxsunOCTControl_LW_C.h:36
AxErr axFirmwareVersion(uint32_t *major, uint32_t *minor, uint32_t *patch, uint32_t which_device)
Gets the device firmware version.
AxErr axGetBoolSetting(uint32_t which_setting, uint8_t *setting_value, char *setting_string, uint32_t which_device)
Gets a boolean setting's value and name string.
@ LASER
Definition: AxsunOCTControl_LW_C.h:44
AxErr axSetEightBitGain(float gain, uint32_t which_DAQ)
Set the GAIN term during 16- to 8-bit dynamic range compression.
AxErr axGetFloatSetting(uint32_t which_setting, float *setting_value, char *setting_string, uint32_t which_device)
Gets a floating point setting's value and name string.
AxErr axSetPointerEmission(uint32_t emission_state, uint32_t which_laser)
Enables or disables pointer/aiming laser emission.
AxErr axConnectionHeartbeat(uint32_t heartbeat_state, uint32_t which_device)
Disable or re-enable the Connection Heartbeat for a device connected by Ethernet network or RS-232.
AxChannelMode
DAQ channel selection modes.
Definition: AxsunCommonEnums.h:211
@ ETHERNET
Definition: AxsunOCTControl_LW_C.h:39
uint32_t axCountConnectedDevices()
Counts the number of devices successfully connected and enumerated by AxsunOCTControl.
@ RS232_PASSTHROUGH
Definition: AxsunOCTControl_LW_C.h:37
AxErr axRegisterLabVIEWCallback(LVUserEventRef *refnumptr)
Registers a LabVIEW user event to be executed following a device connection or disconnection event.
@ ERROR_WENT_OUT_OF_RANGE
Definition: AxsunOCTControl_LW_C.h:57
AxErr axSetImageSyncSource(AxEdgeSource source, float frequency, uint32_t which_DAQ)
Select the Image_sync source.
AxErr axLibraryVersion(uint32_t *major, uint32_t *minor, uint32_t *patch, uint32_t *build)
Gets the library version.
void(__cdecl * AxConnectCallbackFunctionC_t)(void *)
Type defintion for a user-provided function to be called upon connection or disconnection of a device...
Definition: AxsunOCTControl_LW_C.h:61
AxErr axGetPointerEmission(uint32_t *emitting, uint32_t which_laser)
Gets pointer/aiminglaser emission status.
AxErr axGetTECState(AxTECState *TEC_state, uint32_t which_TEC, uint32_t which_laser)
Gets the current state of a Laser Thermo-Electric Cooler (TEC).
AxErr axGetFPGARegisterRange(const uint32_t start_regnum, const uint32_t end_regnum, uint16_t *regvals, const uint32_t bytes_allocated, uint32_t which_DAQ)
Gets (i.e. reads) current values in a range of FPGA registers.
@ READY
Definition: AxsunOCTControl_LW_C.h:54
AxErr axMoveRelVDL(float rel_position, float speed, uint32_t which_laser)
Move the VDL relative to its current position.
@ LVDS
Definition: AxsunOCTControl_LW_C.h:30
@ RS232
Definition: AxsunOCTControl_LW_C.h:38
AxErr axSerialNumber(char *serial_number, uint32_t which_device)
Gets the device serial number string.
AxEdgeSource
DAQ clock and trigger sources.
Definition: AxsunOCTControl_LW_C.h:27
AxErr axImagingCntrlEthernet(int16_t number_of_images, uint32_t which_DAQ)
Control the DAQ operational mode (Live Imaging, Burst Recording, or Imaging Off) when using the Ether...
AxErr axGetDACTable(uint32_t *points, uint32_t *speed, uint32_t *timer1time, uint32_t *timer2time, uint32_t *timer1duration, uint32_t *timer2duration, uint16_t *volts_currents, uint32_t which_laser)
Gets the currently configured DAC table.
This header file contains enums and other definitions for integrating AxsunOCTCapture....
AxPipelineMode
DAQ pipeline modes.
Definition: AxsunCommonEnums.h:193
AxErr axSetSampleClockSource(AxEdgeSource source, uint32_t which_DAQ)
Select the ADC sample clock source.
AxErr axReadDAQPHYRegister(uint32_t phynum, uint32_t regnum, uint16_t *regval, uint32_t which_DAQ)
Reads the current value in a DAQ PHY register.
AxTECState
Laser TEC states.
Definition: AxsunOCTControl_LW_C.h:50
AxErr axSetClockDelay(uint32_t delay_code, uint32_t which_laser)
Sets the electronic K-clock delay.
AxErr axGetClockDelay(uint32_t *delay_code, uint32_t which_laser)
Gets the currently configured electronic K-clock delay.
AxDevType
Definition: AxsunOCTControl_LW_C.h:42
@ WAITING_IN_RANGE
Definition: AxsunOCTControl_LW_C.h:53
AxErr axSetPipelineMode(AxPipelineMode pipeline_mode, AxChannelMode polarization_mode, uint32_t which_DAQ)
Select the DAQ's pipeline mode (i.e. bypass mode) and polarization channel configuration.
AxErr axCloseAxsunOCTControl()
Closes an AxsunOCTControl context previously opened with axOpenAxsunOCTControl().
AxErr axGetLaserEmission(uint32_t *emitting, uint32_t which_laser)
Gets swept laser emission status.
AxErr axSetFPGARegisterSingleBit(const uint32_t regnum, const uint32_t which_bit, const uint32_t value, uint32_t which_DAQ)
Sets or Clears a single bit in a FPGA register.
AxErr axConnectionType(AxConnectionType *connection_type, uint32_t which_device)
Gets the device connection interface (e.g. USB, Ethernet, RS-232).
AxErr axNetworkInterfaceOpen(uint32_t interface_status)
Opens, resets, or closes the Ethernet network interface.
@ EDAQ
Definition: AxsunOCTControl_LW_C.h:46
@ WARMING_UP
Definition: AxsunOCTControl_LW_C.h:52
AxErr axSetFPGARegisterMasked(const uint32_t regnum, const uint16_t regval, const uint16_t bitmask, uint32_t which_DAQ)
Sets (i.e. writes) FPGA register bits based on a bitmask.
AxErr axHomeVDL(uint32_t which_laser)
Starts the VDL home operation.
AxErr axGetFPGARegisterDefaults(uint32_t elements_allocated, uint16_t *regnums, uint16_t *regvals, uint32_t *elements_returned, uint32_t which_DAQ)
Gets (i.e. reads) the programmed power-on default FPGA register configuration script as register numb...
AxErr axFPGAVersion(uint32_t *major, uint32_t *minor, uint32_t *patch, uint32_t *build, uint32_t which_device)
Gets the device FPGA version.
AxErr axGetDriveConfiguration(uint32_t *current_configuration, uint32_t which_laser)
Gets the currently selected drive configuration.
AxErr axMoveAbsVDL(float abs_position, float speed, uint32_t which_laser)
Move the VDL to an absolute position.
AxErr
Error codes returned from AxsunOCTCapture or AxsunOCTControl_LW functions. Use axGetErrorString() in ...
Definition: AxsunCommonEnums.h:34
AxErr axDebugCommand(uint32_t command_number, uint32_t value_out, uint32_t *command_in, uint32_t *value_in, uint32_t which_device)
Send a debug command.
@ CLDAQ
Definition: AxsunOCTControl_LW_C.h:45
AxErr axSetFPGARegisterDefaults(const uint16_t *regnums, const uint16_t *regvals, const uint32_t count, uint32_t which_DAQ)
Sets (i.e. writes) a power-on default FPGA register configuration script, overwriting the existing sc...
AxErr axSetSweepTriggerSource(AxEdgeSource source, uint32_t which_DAQ)
Select the sweep trigger source.
AxErr axStopVDL(uint32_t which_laser)
Stops any VDL operation (e.g. move or home) currently in progress.
AxErr axDeviceType(AxDevType *device_type, uint32_t which_device)
Gets the device type (e.g. Laser, EDAQ).
@ INTERNAL
Definition: AxsunOCTControl_LW_C.h:29
AxErr axSetLaserEmission(uint32_t emission_state, uint32_t which_laser)
Enables or disables swept laser emission.
AxErr axSetDriveConfiguration(uint32_t which_config, uint32_t which_laser)
Selects a pre-programmed laser drive configuration.
AxErr axSetFPGADataArray(const uint32_t regnum, const uint16_t *data_array, const uint32_t number_of_words, uint32_t which_DAQ)
Sets (i.e. writes) a FPGA register with an array of multiple values.
AxConnectionType
Definition: AxsunOCTControl_LW_C.h:34
AxErr axSetDACTable(uint32_t points, uint32_t speed, uint32_t timer1time, uint32_t timer2time, uint32_t timer1duration, uint32_t timer2duration, uint16_t *volts_currents, uint32_t save_to_flash, uint32_t which_laser)
Sets the DAC table.
AxErr axCountDeviceSettings(uint32_t *float_count, uint32_t *int_count, uint32_t *bool_count, uint32_t which_device)
Counts the number of individual device settings elements for each of the three data types (float,...
void axGetErrorExplained(AxErr errornum, char *error_string)
Gets a string which explains an error code in a more verbose fashion.
AxErr axSetSubsamplingFactor(uint8_t subsampling_factor, uint32_t which_DAQ)
Set the A-line subsampling factor.
AxErr axGetVDLStatus(float *current_pos, float *target_pos, float *speed, int32_t *error_from_last_home, uint32_t *last_move_time, uint8_t *state, uint8_t *home_switch, uint8_t *limit_switch, uint8_t *VDL_error, uint32_t which_laser)
Gets the current status of the VDL.
@ ERROR_NEVER_GOT_TO_READY
Definition: AxsunOCTControl_LW_C.h:56
@ NOT_INSTALLED
Definition: AxsunOCTControl_LW_C.h:55
AxErr axSetEightBitOffset(float offset, uint32_t which_DAQ)
Set the OFFSET term during 16- to 8-bit dynamic range compression.